Archive Catalog Developer Tools
Below are some open source tools commonly used to search and visualize STAC APIs that we recommend considering when looking to search the Umbra Archive Catalog.
Pystac Client
Pystac-client is a library for Python that is used to search a STAC API. To install it:
pip install pystac-client
You can then use it to connect to Archive Catalog and perform a search. Don't forget to add your Auth Token .
import pystac_client
CANOPY_TOKEN="..."
stac_api_url = "https://api.canopy.umbra.space/archive"
catalog = pystac_client.Client.open(stac_api_url, headers={
'Authorization': f"Bearer {CANOPY_TOKEN}"
})
time_range = "2020-01-01/2024-12-31"
# You can create a supported geometry using https://geojson.io/
intersect_geometry = None
stac_search = catalog.search(max_items=10, collections=["umbra-sar"], intersects=intersect_geometry, datetime=time_range)
items = stac_search.item_collection()
print(items[0].to_dict())
items
You can use https://geojson.io/ to create an intersect_geometry by copying over the geometry dictionary and assigning it to intersect_geometry
.
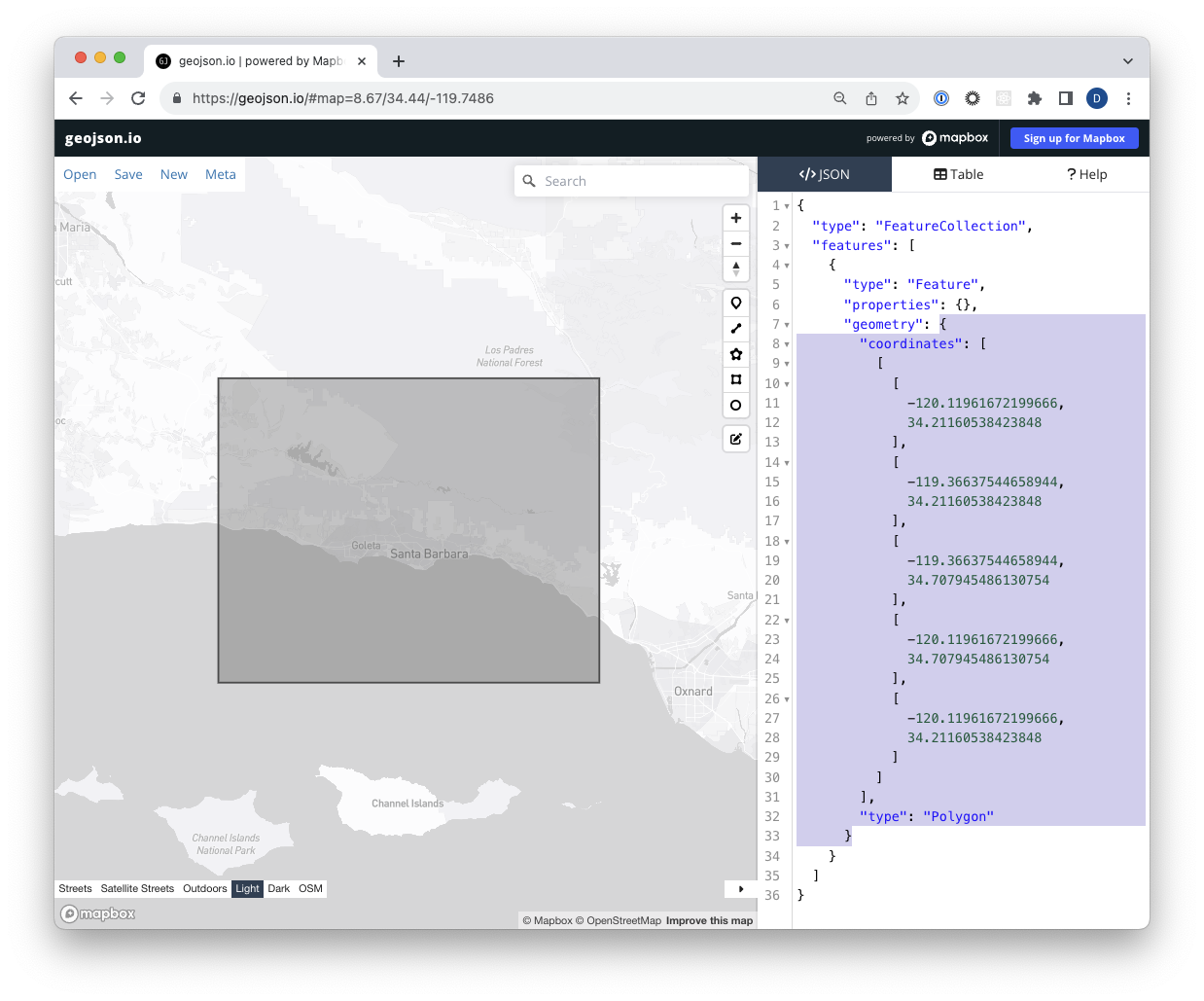
If you are using Jupyter, thumbnails from the STAC items can be displayed in the Notebook.
from IPython.display import Image
def display_item_thumbnail(item):
return Image(url=item.assets['thumbnail'].href)
display_item_thumbnail(items[0])
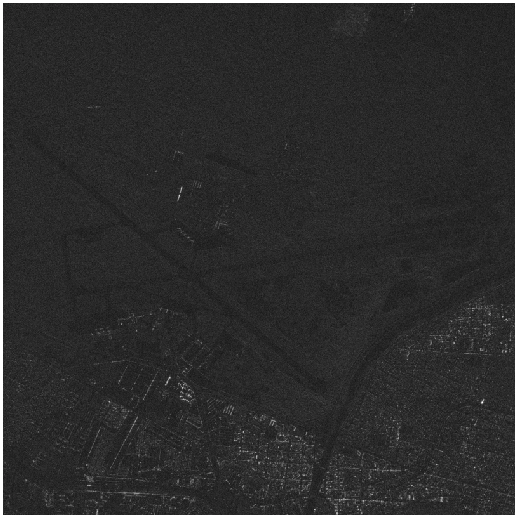
Leafmap
Leafmap is a Python package for searching and visualizing geospatial data with an interactive map in Jupyter. Start by installing it:
pip install leafmap
Then use it inside a Jupyter Notebook to display an interactive map:
import leafmap
search_map = leafmap.Map(zoom=4)
search_map
You can draw a box on the map to use as bounds for a STAC API search.
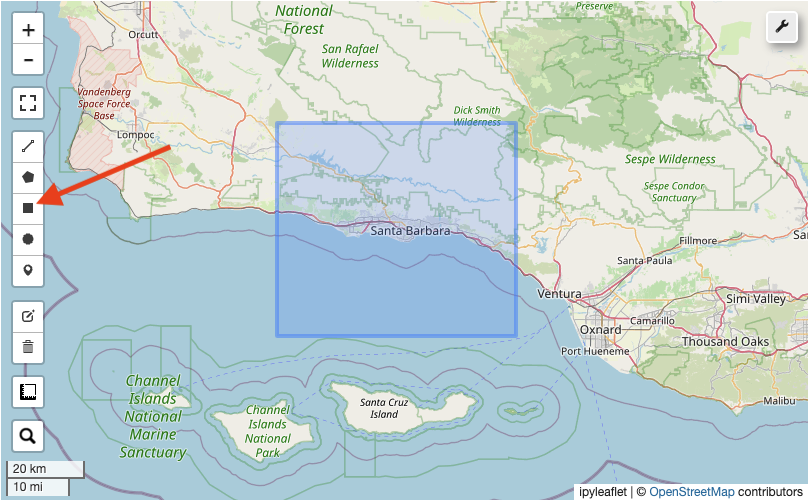
After drawing the box, send a search request.
if len(m.draw_features) == 1:
intersects = m.draw_features[0]['geometry']
bbox = None
print("You have drawn a Feature on Leafmap. Using your selection to perform the search.")
else:
intersects = None
bbox = m.get_bbox()
print("You have not drawn anything on the Leafmap. Using the entire bounds to perform the search.")
stac_api_url = "https://api.canopy.umbra.space/archive"
time_range = "2020-01-01/2024-12-31"
def inject_auth(request):
request.headers["Authorization"] = f"Bearer {CANOPY_TOKEN}"
return request
items = leafmap.stac_search(
url=stac_api_url,
max_items=10,
collections=["umbra-sar"],
datetime=time_range,
bbox=bbox,
intersects=intersects,
get_collection=True,
requests_callable=inject_auth
)
print(f"Returned {len(items)} results")
And examine the results.
# You have drawn a Feature on Leafmap. Using your selection to perform the search.
# Returned 2 results
print(items[0].to_dict())
items[0]
You can overlay the resulting images on Leafmap.
def layer_from_item(item):
thumbnail_url = item.assets['thumbnail'].href
bbox = item.bbox
bounds = ((bbox[1], bbox[0]), (bbox[3], bbox[2]))
return leafmap.ImageOverlay(
url=thumbnail_url,
bounds=bounds
)
m = leafmap.Map()
for i, item in enumerate(items):
layer = layer_from_item(item)
layer.name = str(i)
m.add_layer(layer)
center = leafmap.get_center(items[0].geometry)
m.set_center(lon=center[0], lat=center[1], zoom=12)
m
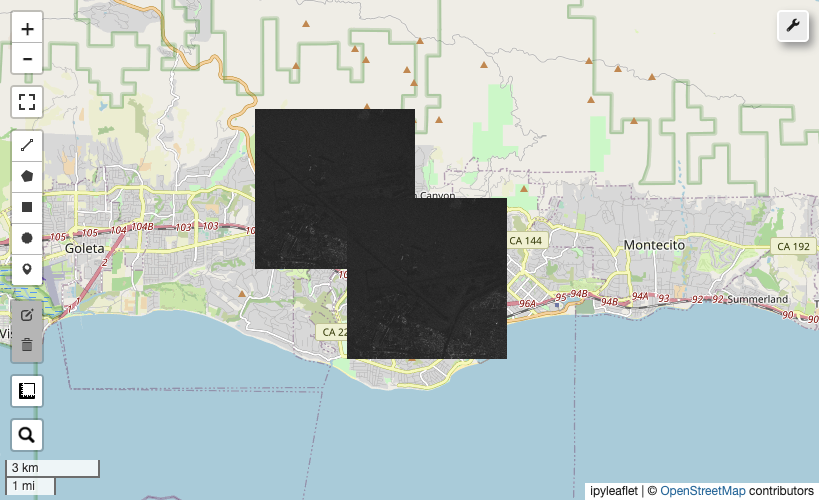
Updated 2 months ago